Fast, flexible, secure backend for your web, mobile, and AI apps
Exograph is a powerful data modeling language and high-performance query engine that offers dynamically generated APIs
- Model relational and vector data
- First-class Postgres integration
- Fine-grained access control
- Query execution in < 20ms
- Small memory footprint < 32MB
- GraphQL APIs (REST & RPC coming soon)
- Custom functions and interceptors
- Deploy to AWS Lambda, Google Cloud, Fly.io & more
- Data Models in Seconds
- Fine-Grained Access Controls
- Connect to Custom Logic
Describe your domain model, and Exograph will:
- Automatically infer GraphQL API for it.
- Create (and migrate) database schema for it.
- Execute queries efficiently
Go beyond role-based authorization. Specify complex permissions with just a few lines of code.
Integrate with any authentication system including OpenID Connect.
context AuthContext {
@jwt role: String
}
@postgres
module EcommerceDatabase {
@access(
query=self.published || AuthContext.role=="admin",
mutation=AuthContext.role=="admin")
type Product {
// ...
published: Boolean
}
@access(
query=true,
mutation=AuthContext.role=="admin")
type Department {
// ...
published: Boolean
}
}
Integrate with external APIs or define custom algorithms with just a few lines of JavaScript or TypeScript.
@deno("announce.ts")
module ProductAnnouncement {
@access(AuthContext.role=="admin")
mutation announce(productId: Int, @inject exo: Exograph): String
}
const productQuery =
`query getProduct($id: Int) {
product(id: $id) {
name,
price
}
}`;
export function announce(productId: number, exo: Exograph): string {
const product = exo.executeQuery(productQuery, {id: productId});
const potentialBuyers = exo.executeQuery(... analytics GraphQL query);
sendEmail(
potentialBuyers,
"New Product", `A new product ${product.name} is available`
);
return "Announcement sent";
}
Deploy Anywhere
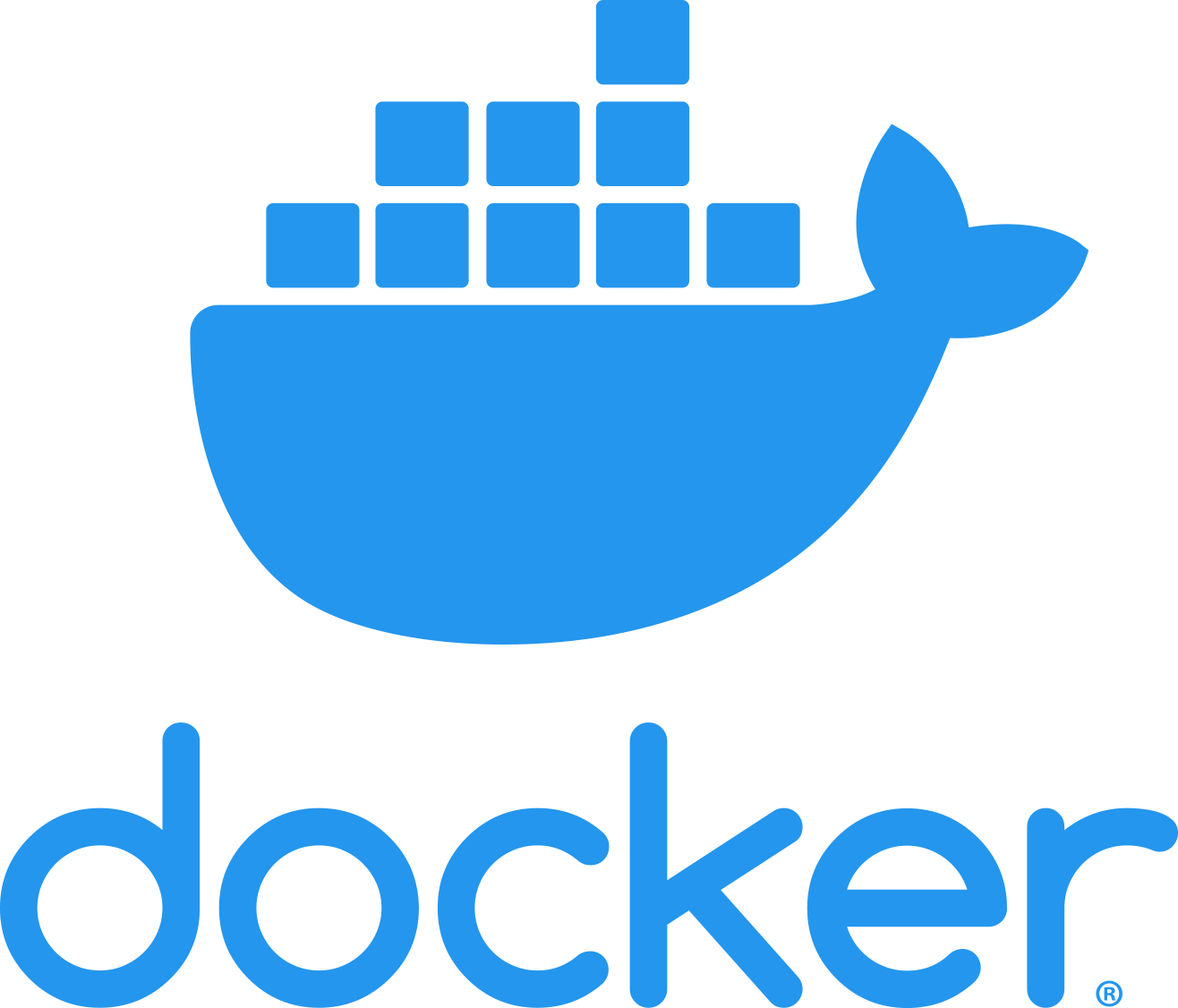
Integrates With
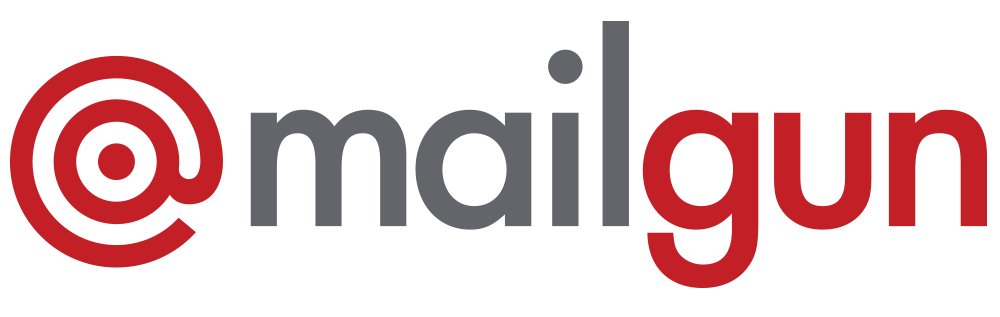
Awesome Developer Experience
Iterate fast with support for schema creation, migration, deployment, and more throughout the application lifecycle.
Exograph models fit right into your existing Git workflow.
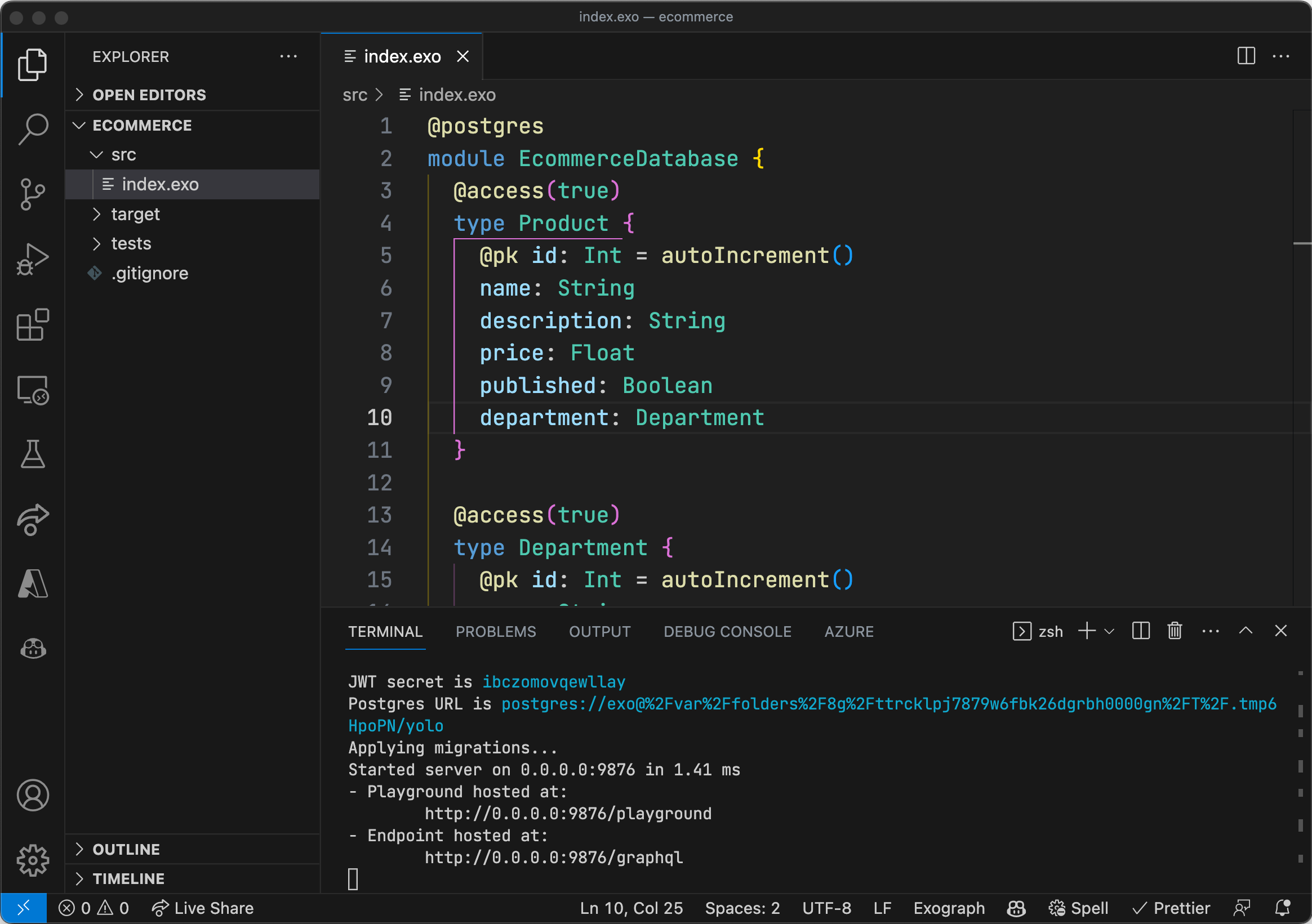